A(N) ____ Read Is Used to Prepare or Set Up a Loop.
1.3 Conditionals and Loops
In the programs that we have examined to this point, each of the statements is executed once, in the order given. Most programs are more complicated because the sequence of statements and the number of times each is executed can vary. We use the term command flow to refer to statement sequencing in a program.
If statements.
Most computations require unlike deportment for different inputs.
- The following code fragment uses an if statement to put the smaller of ii int values in x and the larger of the two values in y, by exchanging the values in the two variables if necessary.
- Flip.java uses Math.random() and an if-else statement to print the results of a coin flip.
- The table below summarizes some typical situations where you might need to utilise an if or if-else argument.
While loops.
Many computations are inherently repetitive. The
whileloop enables us to execute a group of statements many times. This enables usa to express lengthy computations without writing lots of code.
- The post-obit code fragment computes the largest ability of 2 that is less than or equal to a given positive integer n.
- TenHellos.java prints "Hello World" 10 times.
- PowersOfTwo.coffee takes an integer command-line argument n and prints all of the powers of 2 less than or equal to due north.
For loops.
The for loop is an alternate Java construct that allows united states even more flexibility when writing loops.
- For notation. Many loops follow the same basic scheme: initialize an index variable to some value and and then utilize a while loop to examination an exit condition involving the alphabetize variable, using the terminal argument in the while loop to alter the alphabetize variable. Java'south for loop is a direct way to limited such loops.
- Compound assignment idioms. The idiom i++ is a shorthand notation for i = i + 1.
- Scope. The scope of a variable is the part of the program that tin refer to that variable by proper name. Generally the scope of a variable comprises the statements that follow the declaration in the same block as the proclamation. For this purpose, the code in the for loop header is considered to exist in the same block equally the for loop torso.
Nesting.
The
if,
while, and
forstatements have the same status every bit assignment statements or any other statements in Java; that is, we can use them wherever a statement is called for. In particular, nosotros can use one or more of them in the body of some other statement to make compound statements. To emphasize the nesting, we use indentation in the program code.
- DivisorPattern.java has a for loop whose body contains a for loop (whose trunk is an if-else argument) and a print statement. It prints a design of asterisks where the ith row has an asterisk in each position corresponding to divisors of i (the aforementioned holds true for the columns).
- MarginalTaxRate.java computes the marginal revenue enhancement rate for a given income. It uses several nested if-else statements to examination from among a number of mutually exclusive possibilities.
Loop examples.
Applications.
The ability to plan with loops and conditionals immediately opens upward the earth of ciphering to us.
- Ruler subdivisions. RulerN.coffee takes an integer command-line statement n and prints the string of ruler subdivision lengths. This program illustrates i of the essential characteristics of loops—the program could hardly exist simpler, only it can produce a huge corporeality of output.
- Finite sums. The computational paradigm used in PowersOfTwo.java is one that you will use ofttimes. It uses two variables—1 as an index that controls a loop, and the other to accumulate a computational issue. Program HarmonicNumber.java uses the same image to evaluate the sum
$$ H_n = \frac{1}{ane} + \frac{1}{2} + \frac{1}{3} + \frac{1}{4} + \; \ldots \; + \frac{ane}{n} $$
These numbers, which are known as the harmonic numbers, arise frequently in the analysis of algorithms.
- Newton's method.
Sqrt.java uses a archetype iterative technique known equally Newton's method to compute the square root of a positive number x: Start with an estimate t. If t is equal to x/t (up to machine precision), then t is equal to a square root of x, so the computation is complete. If non, refine the approximate by replacing t with the boilerplate of t and x/t. Each time we perform this update, we get closer to the desired reply.
- Number conversion. Binary.java prints the binary (base of operations 2) representation of the decimal number typed every bit the command-line statement. Information technology is based on decomposing the number into a sum of powers of 2. For case, the binary representation of 106 is 1101010two, which is the aforementioned as saying that 106 = 64 + 32 + viii + 2. To compute the binary representation of northward, we consider the powers of two less than or equal to n in decreasing gild to determine which belong in the binary decomposition (and therefore correspond to a 1 bit in the binary representation).
- Gambler'south ruin.
Suppose a gambler makes a serial of off-white $ane bets, starting with $50, and continue to play until she either goes broke or has $250. What are the chances that she will go habitation with $250, and how many bets might she expect to brand earlier winning or losing? Gambler.java is a simulation that tin help answer these questions. It takes iii control-line arguments, the initial stake ($l), the goal amount ($250), and the number of times nosotros want to simulate the game.
- Prime number factorization. Factors.coffee takes an integer control-line argument n and prints its prime factorization. In contrast to many of the other programs that we have seen (which we could do in a few minutes with a calculator or pencil and paper), this ciphering would not be feasible without a computer.
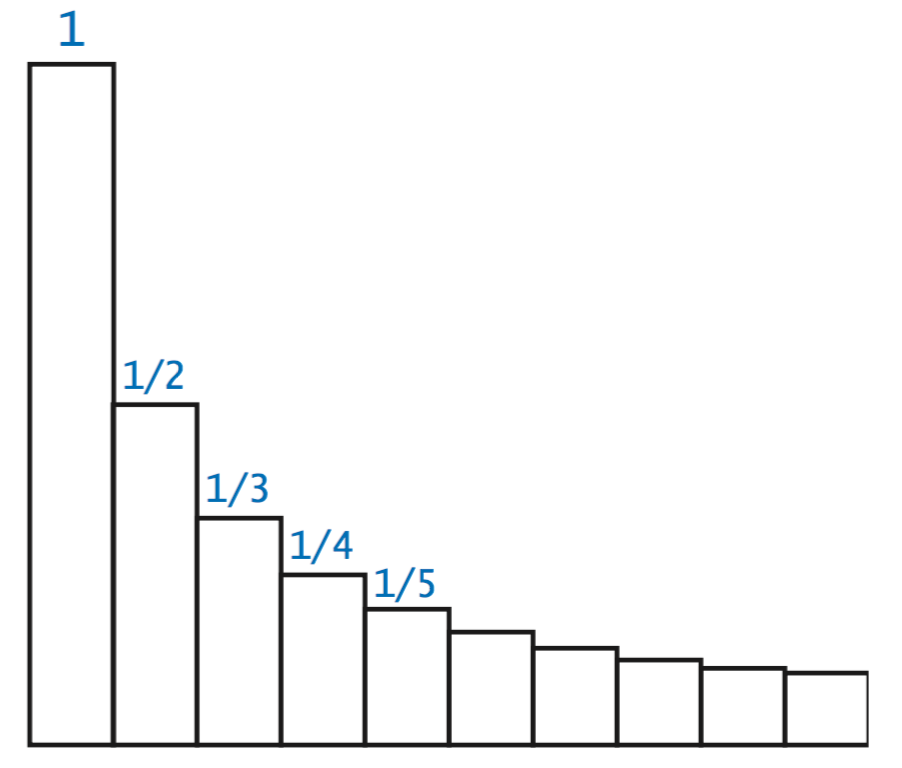
Other conditional and loop constructs.
To be consummate, we consider iv more Java constructs related to conditionals and loops. They are used much less ofttimes than the
if,
while, and
forstatements that we've been working with, but it is worthwhile to be aware of them.
- Break statements. In some situations, we want to immediate exit a loop without letting it run to completion. Java provides the break statement for this purpose. Prime.coffee takes an integer command-line argument n and prints true if n is prime number, and false otherwise. There are 2 different ways to leave this loop: either the break statement is executed (because n is not prime) or the loop-continuation condition is not satisfied (considering due north is prime number).
Annotation that the break argument does non apply to if or if-else statements. In a famous programming bug, the U.S. telephone network crashed because a programmer intended to use a break statement to exit a complicated if statement.
- Go along statements. Java as well provides a style to skip to the adjacent iteration of a loop: the continue statement. When a continue is executed inside the torso of a for loopy, the catamenia of control transfers straight to the increment statement for the next iteration of the loop.
- Switch statements. The if and if-else statements permit one or ii alternatives. Sometimes, a ciphering naturally suggests more than than two mutually exclusive alternatives. Java provides the switch argument for this purpose. NameOfDay.java takes an integer between 0 and 6 as a command-line argument and uses a switch statement to print the corresponding name of the day (Sunday to Saturday).
- Do–while loops. A exercise-while loop is well-nigh the same as a while loop except that the loop-continuation condition is omitted the commencement time through the loop. RandomPointInCircle.coffee sets 10 and y and then that (x, y) is randomly distributed within the circle centered at (0, 0) with radius 1.
With Math.random() we get points that are randomly distributed in the two-by-2 foursquare eye at (0, 0). We simply generate points in this region until we detect one that lies within the unit disk. We always desire to generate at to the lowest degree ane point and then a do-while loop is nearly appropriate. We must declare x and y exterior the loop since we will want to access their values after the loop terminates.
We don't use the following two menstruum command statements in this textbook, but include them here for abyss.
- Conditional operator. The conditional operator ?: is a ternary operator (iii operands) that enables you to embed a conditional within an expression. The iii operands are separated past the ? and : symbols. If the first operand (a boolean expression) is true, the result has the value of the second expression; otherwise it has the value of the third expression.
int min = (x < y) ? x : y;
- Labeled interruption and go along statements. The suspension and go along statements apply to the innermost for or while loop. Sometimes we want to jump out of several levels of nested loops. Java provides the labeled break and labeled continue statements to accomplish this. Here is an case.
Exercises
- Write a program AllEqual.coffee that takes three integer command-line arguments and prints equal if all iii are equal, and not equal otherwise.
- Write a program RollLoadedDie.java that prints the result of rolling a loaded die such that the probability of getting a 1, 2, iii, 4, or 5 is 1/8 and the probability of getting a 6 is three/8.
- Rewrite TenHellos.java to make a plan Hellos.java that takes the number of lines to print as a command-line argument. You may assume that the argument is less than 1000. Hint: consider using i % 10 and i % 100 to determine whether to use "st", "nd", "rd", or "th" for printing the ith Hello.
- Write a program FivePerLine.coffee that, using 1 for loop and one if statement, prints the integers from thou to 2000 with v integers per line. Hint: utilise the % operator.
- Write a program FunctionGrowth.java that prints a table of the values of ln n, north, n ln n, north2 , nthree , and 2north for n = 16, 32, 64, ..., 2048. Use tabs ('\t' characters) to line upward columns.
- What is the value of thousand and n after executing the post-obit lawmaking?
int n = 123456789; int grand = 0; while (n != 0) { grand = (10 * g) + (n % 10); n = n / 10; }
- What does the following code print out?
int f = 0, yard = ane; for (int i = 0; i <= 15; i++) { System.out.println(f); f = f + g; grand = f - g; }
- Different the harmonic numbers, the sum one/1 + 1/iv + ane/nine + ane/16 + ... + 1/northii does converge to a abiding as north grows to infinity. (Indeed, the abiding is πtwo / vi, then this formula can be used to judge the value of π.) Which of the post-obit for loops computes this sum? Assume that n is an int initialized to one thousand thousand and sum is a double initialized to 0.
(a) for (int i = ane; i <= north; i++) sum = sum + 1 / (i * i); (b) for (int i = 1; i <= n; i++) sum = sum + 1.0 / i * i; (c) for (int i = 1; i <= n; i++) sum = sum + 1.0 / (i * i); (d) for (int i = 1; i <= northward; i++) sum = sum + 1 / (1.0 * i * i);
- Alter Binary.coffee to become a program Change Kary.java that takes a 2d command-line argument One thousand and converts the first statement to base Chiliad. Presume the base is between 2 and xvi. For bases greater than 10, utilize the messages A through F to represent the 11th through 16th digits, respectively.
- Write a program code fragment that puts the binary representation of a positive integer n into a String variable s.
Creative Exercises
- Ramanujan'due south taxi. Due south. Ramanujan was an Indian mathematician who became famous for his intuition for numbers. When the English mathematician G. H. Hardy came to visit him in the hospital 1 day, Hardy remarked that the number of his taxi was 1729, a rather boring number. To which Ramanujan replied, "No, Hardy! No, Hardy! It is a very interesting number. It is the smallest number expressible as the sum of two cubes in ii different ways." Verify this claim by writing a plan Ramanujan.java that takes an integer command-line argument northward and prints all integers less than or equal to north that tin exist expressed every bit the sum of two cubes in ii different ways - find distinct positive integers a, b, c, and d such that a3 + b3 = c3 + d3 . Use iv nested for loops.
Now, the license plate 87539319 seems similar a rather irksome number. Determine why information technology'south not.
- Checksums. The International Standard Book Number (ISBN) is a 10 digit code that uniquely specifies a volume. The rightmost digit is a checksum digit which can be uniquely determined from the other 9 digits from the condition that d1 + second2 + 3dthree + ... + 10d10 must be a multiple of 11 (hither di denotes the ith digit from the right). The checksum digit d1 tin exist whatever value from 0 to ten: the ISBN convention is to employ the value X to denote 10. Example: the checksum digit corresponding to 020131452 is 5 since is the only value of d1 between 0 and and 10 for which d1 + 2*2 + iii*5 + 4*4 + 5*one + half dozen*3 + seven*1 + viii*0 + nine*2 + 10*0 is a multiple of 11. Write a programme ISBN.java that takes a 9-digit integer equally a control-line argument, computes the checksum, and prints the x-digit ISBN number. Information technology'south ok if you don't print whatsoever leading 0s.
- Exponential office. Assume that x is a positive variable of type double. Write a program Exp.java that computes e^ten using the Taylor series expansion
$$ east^ 10 = 1 + x + \frac{x^2}{2!} + \frac{10^3}{3!} + \frac{x^4}{4!} + \ldots $$
- Trigonometric functions. Write two programs Sin.java and Cos.java that compute sin x and cos x using the Taylor series expansions
$$ \sin 10 = ten - \frac{x^iii}{3!} + \frac{x^5}{5!} - \frac{10^7}{7!} + \ldots $$
$$ \cos x = ane - \frac{x^2}{2!} + \frac{x^iv}{4!} - \frac{ten^vi}{vi!} + \ldots $$
- Game simulation. In the game show Let'south Make a Deal, a contestant is presented with iii doors. Behind 1 door is a valuable prize, backside the other two are gag gifts. Later on the contestant chooses a door, the host opens upwards one of the other two doors (never revealing the prize, of form). The contestant is then given the opportunity to switch to the other unopened door. Should the contestant practice so? Intuitively, it might seem that the contestant's initial selection door and the other unopened door are equally likely to comprise the prize, so there would be no incentive to switch. Write a plan MonteHall.java to exam this intuition by simulation. Your program should have an integer control-line argument n, play the game n times using each of the ii strategies (switch or don't switch) and print the chance of success for each strategy. Or you can play the game hither.
- Euler's sum-of-powers conjecture. In 1769 Leonhard Euler formulated a generalized version of Fermat'southward Last Theorem, conjecturing that at least n nth powers are needed to obtain a sum that is itself an due northth power, for north > 2. Write a program Euler.java to disprove Euler's conjecture (which stood until 1967), using a quintuply nested loop to detect iv positive integers whose 5th power sums to the 5th power of another positive integer. That is, observe a, b, c, d, and e such that a 5 + b 5 + c 5 + d 5 = e 5. Employ the long data blazon.
Web Exercises
- Write a program RollDie.java that generates the upshot of rolling a fair six-sided die (an integer between 1 and 6).
- Write a program that takes three integer command-line arguments a, b, and c and print the number of distinct values (1, 2, or three) amid a, b, and c.
- Write a program that takes five integer command-line arguments and prints the median (the third largest one).
- (difficult) Now, attempt to compute the median of 5 elements such that when executed, it never makes more than 6 total comparisons.
- How can I create in an infinite loop with a for loop?
Solution: for(;;) is the same every bit while(truthful).
- What's wrong with the following loop?
boolean done = fake; while (done = false) { ... }
boolean done = false; while (!done) { ... }
- What's wrong with the following loop that is intended to compute the sum of the integers 1 through 100?
for (int i = one; i <= North; i++) { int sum = 0; sum = sum + i; } System.out.println(sum);
- Write a programme Hurricane.java that that takes the wind speed (in miles per 60 minutes) as an integer command-line statement and prints whether it qualifies every bit a hurricane, and if then, whether it is a Category 1, 2, three, 4, or v hurricane. Below is a table of the wind speeds according to the Saffir-Simpson scale.
Category Wind Speed (mph) 1 74 - 95 2 96 - 110 3 111 - 130 4 131 - 155 5 155 and above - What is wrong with the following code fragment?
double x = -32.two; boolean isPositive = (ten > 0); if (isPositive = true) System.out.println(ten + " is positive"); else System.out.println(x + " is not positive");
Solution: It uses the assignment operator = instead of the equality operator ==. A improve solution is to write if (isPositive).
- Change/add one character so that the following plan prints 20 xs. There are 2 unlike solutions.
int i = 0, n = 20; for (i = 0; i < due north; i--) System.out.print("x");
- What does the following code fragment do?
if (ten > 0); System.out.println("positive");
Solution: always prints positive regardless of the value of x because of the extra semicolon after the if statement.
- RGB to HSB converter. Write a program RGBtoHSV.java that takes an RGB color (3 integers between 0 and 255) and transforms it to an HSB color (iii different integers betwixt 0 and 255). Write a plan HSVtoRGB.coffee that applies the inverse transformation.
- Boys and girls. A couple beginning a family unit decides to keep having children until they accept at least i of either sex. Gauge the average number of children they will have via simulation. As well estimate the near common outcome (tape the frequency counts for 2, 3, and 4 children, and besides for 5 and above). Assume that the probability p of having a boy or girl is 1/two.
- What does the following program do?
public static void main(String[] args) { int N = Integer.parseInt(args[0]); int 10 = 1; while (N >= ane) { System.out.println(x); ten = 2 * x; Due north = N / 2; } }
- Boys and girls. Echo the previous question, but assume the couple keeps having children until they have another child which is of the aforementioned sex as the outset kid. How does your answer change if p is different from ane/two?
Surprisingly, the boilerplate number of children is 2 if p = 0 or 1, and three for all other values of p. Just the nigh probable value is 2 for all values of p.
- Given 2 positive integers a and b, what event does the following code fragment leave in c
c = 0; while (b > 0) { if (b % 2 == one) c = c + a; b = b / 2; a = a + a; }
Solution: a * b.
- Write a program using a loop and iv conditionals to impress
12 midnight 1am 2am ... 12 noon 1pm ... 11pm
- What does the following programme impress?
public grade Exam { public static void main(String[] args) { if (10 > five); else; { System.out.println("Here"); }; } }
- Alice tosses a fair coin until she sees two consecutive heads. Bob tosses another fair coin until he sees a head followed by a tail. Write a program to estimate the probability that Alice will brand fewer tosses than Bob? Solution: 39/121.
- Rewrite DayOfWeek.coffee from Exercise 1.two.29 so that it prints the day of the calendar week as Sun, Monday, and and then forth instead of an integer betwixt 0 and six. Employ a switch statement.
- Number-to-English. Write a program to read in a command line integer between -999,999,999 and 999,999,999 and print the English equivalent. Here is an exhaustive list of words that your program should use: negative, zero, i, two, three, four, v, six, seven, viii, 9, ten, eleven, twelve, thirteen, fourteen, fifteen, sixteen, seventeen, eighteen, xix, 20, thirty, forty, fifty, sixty, seventy, eighty, ninety, hundred, one thousand, million . Don't utilize hundred, when yous tin can use g, e.g., use 1 thousand v hundred instead of xv hundred. Reference.
- Gymnastics judging. A gymnast's score is determined past a console of 6 judges who each decide a score between 0.0 and x.0. The last score is adamant past discarding the loftier and low scores, and averaging the remaining 4. Write a program GymnasticsScorer.java that takes vi existent command line inputs representing the 6 scores and prints their boilerplate, after throwing out the high and low scores.
- Quarterback rating. To compare NFL quarterbacks, the NFL devised a the quarterback rating formula based on the quarterbacks number of completed passes (A), pass attempts (B), passing yards (C), touchdown passes (D), and interception (E) as follows:
- Completion ratio: West = 250/3 * ((A / B) - 0.3).
- Yards per pass: X = 25/half-dozen * ((C / B) - 3).
- Touchdown ratio: Y = 1000/3 * (D / B)
- Interception ratio: Z = 1250/3 * (0.095 - (Due east / B))
- Decimal expansion of rational numbers. Given two integers p and q, the decimal expansion of p/q has an infinitely repeating cycle. For instance, 1/33 = 0.03030303.... We use the notation 0.(03) to announce that 03 repeats indefinitely. Every bit another instance, 8639/70000 = 0.1234(142857). Write a program DecimalExpansion.coffee that reads in ii control line integers p and q and prints the decimal expansion of p/q using the in a higher place notation. Hint: use Floyd's rule.
- Friday the 13th. What is the maximum number of consecutive days in which no Friday the 13th occurs? Hint: The Gregorian calendar repeats itself every 400 years (146097 days) so yous only need to worry near a 400 twelvemonth interval.
Solution: 426 (e.g., from viii/xiii/1999 to 10/13/2000).
- January 1. Is January 1 more likely to autumn on a Saturday or Dominicus? Write a program to make up one's mind the number of times each occurs in a 400 yr interval.
Solution: Sunday (58 times) is more likely than Saturday (56 times).
- What exercise the following two lawmaking fragments do?
for (int i = 0; i < Northward; i++) for (int j = 0; j < N; j++) if (i != j) Arrangement.out.println(i + ", " + j); for (int i = 0; i < N; i++) for (int j = 0; (i != j) && (j < N); j++) System.out.println(i + ", " + j);
- Determine what value gets printed out without using a computer. Choose the right answer from 0, 100, 101, 517, or thou.
int cnt = 0; for (int i = 0; i < 10; i++) for (int j = 0; j < 10; j++) for (int k = 0; thousand < 10; k++) if (ii*i + j >= 3*1000) cnt++; System.out.println(cnt);
- Rewrite CarLoan.java from Artistic Do XYZ and so that it properly handles an interest rate of 0% and avoids dividing by 0.
- Write the shortest Java programme yous can that takes an integer control-line argument n and prints true if (1 + 2 + ... + northward) ii is equal to (13 + 2iii + ... + n3).
Solution: Always impress true.
- Change Sqrt.java so that it reports an error if the user enters a negative number and works properly if the user enters goose egg.
- What happens if nosotros initialize t to -x instead of x in program Sqrt.java?
- Sample standard deviation of uniform distribution. Modify Exercise 8 and so that it prints the sample standard divergence in addition to the boilerplate.
- Sample standard deviation of normal distribution. that takes an integer North every bit a command-line statement and uses Web Practise 1 from Section ane.ii to impress N standard normal random variables, and their average value, and sample standard deviation.
- Loaded dice. [Stephen Rudich] Suppose you accept three, three sided dice. A: {2, six, 7}, B: { one, 5, 9}, and C: {three, four, 8}. Two players roll a die and the ane with the highest value wins. Which dice would you choose? Solution: A beats B with probability 5/9, B beats C with probability 5/9 and C beats A with probability v/nine. Be certain to cull 2nd!
- Thue–Morse sequence. Write a programme ThueMorse.java that reads in a control line integer north and prints the Thue–Morse sequence of order north. The first few strings are 0, 01, 0110, 01101001. Each successive string is obtained past flipping all of the bits of the previous cord and concatenating the effect to the end of the previous cord. The sequence has many amazing properties. For case, it is a binary sequence that is cube-free: information technology does not contain 000, 111, 010101, or sss where southward is any string. It is self-similar: if yous delete every other bit, you get another Thue–Morse sequence. It arises in diverse areas of mathematics besides as chess, graphic blueprint, weaving patterns, and music composition.
- Program Binary.java prints the binary representation of a decimal number n past casting out powers of 2. Write an alternate version Program Binary2.java that is based on the following method: Write 1 if n is odd, 0 if northward is even. Split n by 2, throwing away the remainder. Repeat until n = 0 and read the respond backwards. Use % to make up one's mind whether north is even, and use string concatenation to form the answer in reverse order.
- What does the following code fragment do?
int digits = 0; do { digits++; n = n / 10; } while (n > 0);
Solution: The number of bits in the binary representation of a natural number northward. We use a practice-while loop and then that lawmaking output 1 if due north = 0.
- Write a program NPerLine.java that takes an integer command-line statement n and prints the integers from ten to 99 with n integers per line.
- Modify NPerLine.java so that it prints the integers from one to 1000 with n integers per line. Make the integers line up by printing the right number of spaces before an integer (east.thousand., iii for 1-ix, two for x-99, and i for 100-999).
- Suppose a, b, and c are random number uniformly distributed between 0 and 1. What is the probability that a, b, and c form the side length of some triangle? Hint: they will form a triangle if and just if the sum of every two values is larger than the third.
- Repeat the previous question, but calculate the probability that the resulting triangle is birdbrained, given that the three numbers for a triangle. Hint: the three lengths will form an birdbrained triangle if and only if (i) the sum of every ii values is larger than the third and (ii) the sum of the squares of every ii side lengths is greater than or equal to the square of the 3rd.
Answer.
- What is the value of s after executing the following code?
int Yard = 987654321; String due south = ""; while (M != 0) { int digit = M % x; s = southward + digit; M = Yard / 10; }
- What is the value of i afterward the post-obit confusing code is executed?
int i = 10; i = i++; i = ++i; i = i++ + ++i;
Moral: don't write code like this.
- Formatted ISBN number. Write a program ISBN2.coffee that reads in a 9 digit integer from a command-line argument, computes the check digit, and prints the fully formatted ISBN number, e.g, 0-201-31452-5.
- UPC codes. The Universal Product Code (UPC) is a 12 digit code that uniquely specifies a product. The to the lowest degree pregnant digit d1(rightmost i) is a check digit which is the uniquely determined by making the following expression a multiple of x:
(d1 + d3 + d5 + d7 + dnine + dxi) + three (dtwo + dfour + d6 + dviii + d10 + d12)
As an example, the cheque digit corresponding to 0-48500-00102 (Tropicana Pure Premium Orange Juice) is 8 since
(8 + 0 + 0 + 0 + 5 + 4) + 3 (2 + 1 + 0 + 0 + 8 + 0) = 50
and l is a multiple of 10. Write a program that reads in a 11 digit integer from a command line parameter, computes the check digit, and prints the the full UPC. Hint: use a variable of blazon long to store the 11 digit number.
- Write a program that reads in the wind speed (in knots) equally a command line argument and prints its force according to the Beaufort scale. Use a switch argument.
- Making alter. Write a programme that reads in a control line integer N (number of pennies) and prints the all-time way (fewest number of coins) to make change using U.s. coins (quarters, dimes, nickels, and pennies only). For example, if Due north = 73 then print
2 quarters 2 dimes iii pennies
Hint: use the greedy algorithm. That is, dispense as many quarters as possible, then dimes, then nickels, and finally pennies.
- Write a plan Triangle.coffee that takes a command-line statement N and prints an N-by-N triangular blueprint like the one below.
* * * * * * . * * * * * . . * * * * . . . * * * . . . . * * . . . . . *
- Write a plan Ex.coffee that takes a command-line argument N and prints a (2N + 1)-by-(2N + 1) ex similar the one below. Utilize two for loops and one if-else statement.
* . . . . . * . * . . . * . . . * . * . . . . . * . . . . . * . * . . . * . . . * . * . . . . . *
- Write a program BowTie.java that takes a command-line argument North and prints a (2N + 1)-by-(2N + 1) bowtie similar the ane beneath. Apply two for loops and one if-else statement.
* . . . . . * * * . . . * * * * * . * * * * * * * * * * * * * . * * * * * . . . * * * . . . . . *
- Write a programme Diamond.java that takes a command-line argument N and prints a (2N + 1)-by-(2N + 1) diamond like the one below.
% java Diamond iv . . . . * . . . . . . . * * * . . . . . * * * * * . . . * * * * * * * . * * * * * * * * * . * * * * * * * . . . * * * * * . . . . . * * * . . . . . . . * . . . .
- Write a plan Heart.java that takes a control-line argument N and prints a heart.
- What does the program Circle.java print out when Northward = five?
for (int i = -N; i <= N; i++) { for (int j = -N; j <= Northward; j++) { if (i*i + j*j <= N*Northward) Organisation.out.print("* "); else System.out.impress(". "); } System.out.println(); }
- Seasons. Write a plan Flavour.java that takes two command line integers M and D and prints the flavour corresponding to month Grand (ane = January, 12 = December) and twenty-four hours D in the northern hemisphere. Apply the following tabular array
SEASON FROM TO Spring March 21 June 20 Summer June 21 September 22 Fall September 23 Dec 21 Winter December 21 March xx - Zodiac signs. Write a plan Zodiac.java that takes 2 command line integers M and D and prints the Zodiac sign corresponding to month Thou (1 = January, 12 = December) and day D. Apply the following table
SIGN FROM TO Capricorn December 22 January xix Aquarius January 20 Feb 17 Pisces Feb eighteen March 19 Aries March 20 April 19 Taurus April 20 May 20 Gemini May 21 June 20 Cancer June 21 July 22 Leo July 23 August 22 Virgo Baronial 23 September 22 Libra September 23 Oct 22 Scorpio October 23 November 21 Sagittarius Nov 22 December 21 - Muay Thai kickboxing. Write a program that reads in the weight of a Muay Thai kickboxer (in pounds) every bit a command-line argument and prints their weight grade. Use a switch statement.
Course FROM TO Flyweight 0 112 Super flyweight 112 115 Bantamweight 115 118 Super bantamweight 118 122 Featherweight 122 126 Super featherweight 126 130 Lightweight 130 135 Super lightweight 135 140 Welterweight 140 147 Super welterweight 147 154 Middleweight 154 160 Super middleweight 160 167 Light heavyweight 167 175 Super light heavyweight 175 183 Cruiserweight 183 190 Heavyweight 190 220 Super heavyweight 220 - - Euler'southward sum of powers conjecture. In 1769 Euler generalized Fermat's Final Theorem and conjectured that it is incommunicable to find three 4th powers whose sum is a fourth power, or iv 5th powers whose sum is a fifth power, etc. The theorize was disproved in 1966 by exhaustive computer search. Disprove the conjecture by finding positive integers a, b, c, d, and e such that a5 + bv + c5 + d5= e5. Write a plan Euler.coffee that reads in a command line parameter N and exhaustively searches for all such solutions with a, b, c, d, and e less than or equal to N. No counterexamples are known for powers greater than five, but yous can join EulerNet, a distributed computing effort to find a counterexample for sixth powers.
- Blackjack. Write a plan Blackjack.java that takes three command line integers ten, y, and z representing your ii blackjack cards ten and y, and the dealers face-up menu z, and prints the "standard strategy" for a 6 card deck in Atlantic city. Presume that x, y, and z are integers between 1 and 10, representing an ace through a face card. Written report whether the histrion should hit, stand, or split according to these strategy tables. (When you learn virtually arrays, you volition meet an alternating strategy that does non involve every bit many if-else statements).
- Blackjack with doubling. Modify the previous exercise to allow doubling.
- Projectile motion. The following equation gives the trajectory of a ballistic missile as a function of the initial angle theta and windspeed: xxxx. Write a java program to print the (x, y) position of the missile at each fourth dimension stride t. Use trial and error to determine at what angle you should aim the missile if you lot hope to incinerate a target located 100 miles due e of your current location and at the same elevation. Presume the windspeed is 20 mph e.
- World serial. The baseball world series is a best of 7 competition, where the first team to win four games wins the World Series. Suppose the stronger squad has probability p > 1/2 of winning each game. Write a program to guess the chance that the weaker teams wins the World Series and to estimate how many games on boilerplate it will have.
- Consider the equation (9/4)^ten = 10^(9/four). One solution is ix/iv. Tin can yous detect some other one using Newton's method?
- Sorting networks. Write a program Sort3.java with three if statements (and no loops) that reads in 3 integers a, b, and c from the command line and prints them out in ascending order.
if (a > b) swap a and b if (a > c) bandy a and c if (b > c) bandy b and c
- Oblivious sorting network. Convince yourself that the post-obit code fragment rearranges the integers stored in the variables A, B, C, and D so that A <= B <= C <= D.
if (A > B) { t = A; A = B; B = t; } if (B > C) { t = B; B = C; C = t; } if (A > B) { t = A; A = B; B = t; } if (C > D) { t = C; C = D; D = t; } if (B > C) { t = B; B = C; C = t; } if (A > B) { t = A; A = B; B = t; } if (D > E) { t = D; D = E; E = t; } if (C > D) { t = C; C = D; D = t; } if (B > C) { t = B; B = C; C = t; } if (A > B) { t = A; A = B; B = t; }
- Optimal oblivious sorting networks. Create a program that sorts four integers using merely v if statements, and 1 that sorts five integers using only 9 if statements of the type above? Oblivious sorting networks are useful for implementing sorting algorithms in hardware. How can you bank check that your program works for all inputs?
Solution: Sort4.coffee sorts 4 elements using 5 compare-exchanges. Sort5.java sorts v elements using 9 compare-exchanges.
The 0-1 principle asserts that you can verify the correctness of a (deterministic) sorting algorithm by checking whether it correctly sorts an input that is a sequence of 0s and 1s. Thus, to cheque that Sort5.java works, y'all only demand to test it on the two^v = 32 possible inputs of 0s and 1s.
- Optimal oblivious sorting (challenging). Observe an optimal sorting network for 6, 7, and 8 inputs, using 12, xvi, and 19 if statements of the form in the previous problem, respectively.
Solution: Sort6.java is the solution for sorting 6 elements.
- Optimal non-oblivious sorting. Write a program that sorts 5 inputs using only seven comparisons. Hint: Get-go compare the commencement 2 numbers, the 2d ii numbers, and the larger of the two groups, and characterization them so that a < b < d and c < d. Second, insert the remaining chemical element e into its proper identify in the chain a < b < d by first comparing against b, then either a or d depending on the outcome. Third, insert c into the proper place in the chain involving a, b, d, and eastward in the aforementioned style that yous inserted east (with the cognition that c < d). This uses iii (first step) + 2 (second footstep) + two (third step) = 7 comparisons. This method was commencement discovered by H. B. Demuth in 1956.
- Weather balloon. (Etter and Ingber, p. 123) Suppose that h(t) = 0.12t4 + 12t3 - 380t2 + 4100t + 220 represents the peak of a weather balloon at time t (measured in hours) for the first 48 hours after its launch. Create a table of the height at fourth dimension t for t = 0 to 48. What is its maximum height? Solution: t = 5.
- Will the following lawmaking fragment compile? If so, what will it do?
int a = 10, b = 18; if (a = b) System.out.println("equal"); else Organisation.out.println("not equal");
Solution: Information technology uses the consignment operator = instead of the equality operator == in the provisional. In Java, the result of this argument is an integer, but the compiler expects a boolean. As a consequence, the program will not compile. In some languages (notably C and C++), this code fragment will set the variable a to 18 and print equal without an fault.
- Gotcha 1. What does the following lawmaking fragment exercise?
boolean a = faux; if (a = true) Organisation.out.println("yes"); else System.out.println("no");
- Gotcha two. What does the following code fragment do?
int a = 17, x = 5, y = 12; if (x > y); { a = 13; x = 23; } System.out.println(a);
- Gotcha three. What does the following code fragment do?
for (int ten = 0; x < 100; x += 0.5) { System.out.println(ten); }
0
. The compound consignment statementx += 0.v
is equivalent tox = (int) (x + 0.5)
. - What does the following lawmaking fragment practice?
int income = Integer.parseInt(args[0]); if (income >= 311950) rate = .35; if (income >= 174700) rate = .33; if (income >= 114650) rate = .28; if (income >= 47450) rate = .25; if (income >= 0) charge per unit = .22; Organisation.out.println(rate);
- Awarding of Newton's method. Write a plan BohrRadius.java that finds the radii where the probability of finding the electron in the 4s excited state of hydrogen is zero. The probability is given by: (1 - 3r/4 + r2/eight - r3/192)2 e-r/2 , where r is the radius in units of the Bohr radius (0.529173E-8 cm). Use Newton'southward method. By starting Newton's method at unlike values of r, you tin can discover all three roots. Hint: use initial values of r= 0, 5, and 13. Challenge: explain what happens if y'all apply an initial value of r = 4 or 12.
- Pepys problem. In 1693, Samuel Pepys asked Isaac Newton which was more probable: getting at to the lowest degree one ane when rolling a off-white dice 6 times or getting at least two 1'due south when rolling a fair die 12 times. Write a plan Pepys.java that uses simulation to determine the correct answer.
- What is the value of the variable south after running the following loop when N = one, 2, iii, 4, and 5.
String s = ""; for (int i = one; i <= N; i++) { if (i % 2 == 0) s = s + i + s; else s = i + s + i; }
Solution: Palindrome.java.
- Body mass index. The body mass index (BMI) is the ratio of the weight of a person (in kilograms) to the square of the height (in meters). Write a program BMI.java that takes 2 command-line arguments, weight and height, computes the BMI, and prints the respective BMI category:
- Starvation: less than 15
- Anorexic: less than 17.5
- Underweight: less than 18.v
- Platonic: greater than or equal to 18.v but less than 25
- Overweight: greater than or equal to 25 just less than 30
- Obese: greater than or equal to 30 but less than 40
- Morbidly Obese: greater than or equal to 40
- Reynolds number. The Reynolds number is the ratio if inertial forces to viscous forces and is an important quantity in fluid dynamics. Write a program that takes in 4 control-line arguments, the diameter d, the velocity v, the density rho, and the viscosity mu, and prints the Reynold's number d * v * rho / mu (assuming all arguments are in SI units). If the Reynold'due south number is less than 2000, impress laminar flow, if information technology'south betwixt 2000 and 4000, print transient flow, and if it'southward more than 4000, print turbulent menses.
- Wind arctic revisited. The current of air chill formula from Do 1.two.14 is only valid if the wind speed is in a higher place 3MPH and below 110MPH and the temperature is below 50 degrees Fahrenheit and above -l degrees. Modify your solution to print an mistake message if the user types in a value exterior the allowable range.
- Point on a sphere. Write a plan to print the (x, y, z) coordinates of a random point on the surface of a sphere. Use Marsaglia' method: choice a random point (a, b) in the unit circle equally in the practise-while example. Then, set x = 2a sqrt(1 - a^2 - b^2), y = 2b sqrt(1 - a^2 - b^two), z = one - 2(a^2 + b^2).
- Powers of k. Write a program PowersOfK.coffee that takes an integer Thousand as command-line argument and prints all the positive powers of K in the Java long data blazon. Annotation: the abiding Long.MAX_VALUE is the value of the largest integer in long.
- Square root, revisited. Why not apply the loop-continuation condition (Math.abs(t*t - c) > EPSILON) in Sqrt.java instead of Math.abs(t - c/t) > t*EPSILON)?
Solution: Surprisingly, it can lead to inaccurate results or worse. For instance, if yous supply SqrtBug.java with the control-line argument 1e-50, you lot get 1e-50 equally the respond (instead of 1e-25); if you supply 16664444, you get an infinite loop!
- What happens when you try to compile the following code fragment?
double x; if (a >= 0) ten = 3.fourteen; if (a < 0) x = two.71; Arrangement.out.println(x);
Solution: It complains that the variable x might not accept been initialized (even though we can clearly see that ten volition exist initialized by i of the ii if statements). You tin avoid this trouble here by using if-else.
Source: https://introcs.cs.princeton.edu/13flow
Enviar um comentário for "A(N) ____ Read Is Used to Prepare or Set Up a Loop."